Collection framework is use for storing data in program. Collection framework contains most of data structure like LinkedList, Set, HashMap etc already implemented for you to direct use in program and also gives you flexibility to write own kind of data structure.
Java collections framework data structure:
- Lists: Contains list of objects. Java provides 3 type of ready to use list.
- ArrayList
- Vector
- LinkedList
- Sets: Contains unique objects, does not allow dublicates. Java provides 3 type of sets, these are
- HashSet
- LinkedHashSet
- TreeSet
- Maps: Contain key:value data structure, value only access by key. Java provides 4 type of Map.
- HashMap
- Hashtable
- LinkedHashMap
- TreeMap
- Queues: Perform FIFO (first in first out)
- PriorityQueue
Java Collection Interface And Collections Util Class
Collection is an interface which is implemented by all data structure in java, except Map. Maps (HashMap, LinkedHashMap, Hashtable, TreeMap) are not define by Collection interface, they are define by Map interface.Collection Interface
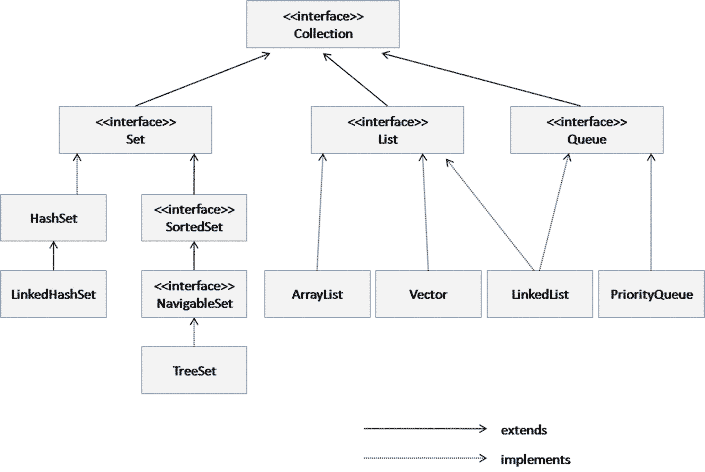
Class and interface structure of maps
Don't confuse between Collection and Collections
- Collection: java.util.Collection is an Interface extends by List, Set, Queue.
- Collections: Collections with 's' java.util.Collections is utility class, which provides many static utility methods to perform on collection(list, set, queue) .
Java List Data Structure
Java List is list of objects, List only care about index. List order by index, means you will get data in which order you would store. In java, we have 3 type of list.- ArrayList: ArrayList is like an array but its growable in size. ArrayList fast in iteration and random access.
- Vector:Vector is same as ArrayList, only one difference, Vector class is thread safe. Its useful when list access by multiple thread at same time.
- LinkedList: LinkedList is different because its elements are doubly linked with each other. It gives you some method like peek(), poll() will helps you to implement other data structure.
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import java.util.Vector;
public class TestList {
public void testArrayList(){
System.out.println("ArrayList");
List list = new ArrayList();
list.add("ola");
list.add("koka");
String str1 = (String) list.get(0);
String str2 = (String) list.get(1);
System.out.println(str1+ " "+str2);
}
public void testVector(){
System.out.println("Vector");
List list = new Vector();
list.add("ola");
list.add("koka");
String str1 = (String) list.get(0);
String str2 = (String) list.get(1);
System.out.println(str1+ " "+str2);
}
public void testLinkedList(){
System.out.println("LinkedList");
List list = new LinkedList();
list.add("ola");
list.add("koka");
String str1 = (String) list.get(0);
String str2 = (String) list.get(1);
System.out.println(str1+ " "+str2);
}
public static void main(String[] args) {
TestList tl = new TestList();
tl.testArrayList();
tl.testVector();
tl.testLinkedList();
}
}
/*
output:
ArrayList
ola koka
Vector
ola koka
LinkedList
ola koka
*/
Java Set Data Structure
Java Set is set of unique object, set don't allow duplicate object to store in Set object. There is three type of set- HashSet: HashSet is an unordered and unsorted data structure collection class. Hence it may possible you will not get data in same sequence as you have inserted data in HashSet.
- LinkedHashSet: It's same as HashSet, not allow duplicate object but LinjkedHashSet is order HashSet which maintain doubly linked between all elements.
- TreeSet: Yes, its not allow duplicate object and TreeSet is sorted collection. It use Red-Black tree structure to sort element of TreeSet.
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.Set;
import java.util.TreeSet;
public class TestSet {
public void testHashSet(){
System.out.println("HashSet");
Set set = new HashSet();
set.add("ola");
set.add("koka");
//dublicate entry
set.add("ola");
//set only access by itterator
Iterator it = set.iterator();
while(it.hasNext()){
System.out.println(it.next());
}
System.out.println("setSize = "+set.size());
}
public void testLinkedHashSet(){
System.out.println("LinkedHashSet");
Set set = new LinkedHashSet();
set.add("ola");
set.add("koka");
//dublicate entry
set.add("ola");
//set only access by itterator
Iterator it = set.iterator();
while(it.hasNext()){
System.out.println(it.next());
}
System.out.println("setSize = "+set.size());
}
public void testTreeSet(){
System.out.println("TreeSet");
Set set = new TreeSet();
set.add("ola");
set.add("koka");
//dublicate entry
set.add("ola");
//set only access by itterator
//Kola print first becauyse TreeSet is sorted collection
Iterator it = set.iterator();
while(it.hasNext()){
System.out.println(it.next());
}
System.out.println("setSize = "+set.size());
}
public static void main(String[] args) {
TestSet ts = new TestSet();
ts.testHashSet();
ts.testLinkedHashSet();
ts.testTreeSet();
}
}
/*
output:
HashSet
koka
ola
setSize = 2
LinkedHashSet
ola
koka
setSize = 2
TreeSet
koka
ola
setSize = 2
*/
Java Map Data Structure
Java Map stores data in Key and Value pair, value get access by key, key must be unique.Java Map Data Structure Types:
- HashMap: HashMap gives you key-value functionality, HashMap is unshorted and unordered collection. HashMap may contain one null key and multiple null values.
- Hashtable: Hashtable is same as HashMap but Hashtable is threadsafe, Hashtable class methods are synchronized. Another difference Hashtable not allow any null in keys or values.
- LinkedHashMap: LinkedHashMap gives you key-value functionality, LinkedHashMap is an ordered collection but not shorted.
- TreeMap: TreeMap is shorted map.
import java.util.HashMap;
import java.util.Hashtable;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.TreeMap;
public class TestMap {
public void testHashMap(){
System.out.println("HashMap");
Map map = new HashMap();
map.put("key1","ola");
map.put("key2","koka");
// we need to cast into String because get method return Object
String v1 = (String) map.get("key1");
String v2 = (String) map.get("key2");
System.out.println( v1 +" and "+ v2);
}
public void testHashtable(){
System.out.println("Hashtable");
Map map = new Hashtable();
map.put("key1","ola");
map.put("key2","koka");
// we need to cast into String because get method return Object
String v1 = (String) map.get("key1");
String v2 = (String) map.get("key2");
System.out.println( v1 +" and "+ v2);
}
public void testLinkedHashMap(){
System.out.println("LinkedHashMap");
Map map = new LinkedHashMap();
map.put("key1","ola");
map.put("key2","koka");
// we need to cast into String because get method return Object
String v1 = (String) map.get("key1");
String v2 = (String) map.get("key2");
System.out.println( v1 +" and "+ v2);
}
public void testTreeMap(){
System.out.println("TreeMap");
Map map = new TreeMap();
map.put("key1","ola");
map.put("key2","koka");
// we need to cast into String because get method return Object
String v1 = (String) map.get("key1");
String v2 = (String) map.get("key2");
System.out.println( v1 +" and "+ v2);
}
public static void main(String[] args) {
TestMap tm = new TestMap();
tm.testHashMap();
tm.testHashtable();
tm.testLinkedHashMap();
tm.testTreeMap();
}
}
/*output:
HashMap
ola and koka
Hashtable
ola and koka
LinkedHashMap
ola and koka
TreeMap
ola and koka
*/
Comments
Post a Comment